Flutter offers Divider widget but it is very simple one and doesn’t offer label. To add label text we need to use Row widget. I created the following widget which can be reused everywhere.
class DividerWithTextWidget extends StatelessWidget {
final String text;
DividerWithTextWidget({required this.text});
@override
Widget build(BuildContext context) {
final line = Expanded(
child: Container(
margin: EdgeInsets.only(left: 10, right: 10),
child: Divider(height: 20, thickness: 5),
));
return Row(children: [line, Text(this.text), line]);
}
}
I used the widget 3 times as follows.
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: ListView(
children: [
DividerWithTextWidget(text: "NAME"),
Padding(child: nameField, padding: EdgeInsets.only(bottom: 20)),
Padding(child: typeRow, padding: EdgeInsets.only(bottom: 20)),
DividerWithTextWidget(text: "UNIT"),
Padding(child: unitRow, padding: EdgeInsets.only(bottom: 20)),
DividerWithTextWidget(text: "CATEGORY"),
Padding(child: categoryRow, padding: EdgeInsets.only(bottom: 20)),
Padding(
child: parentCategoryRow, padding: EdgeInsets.only(bottom: 20)),
_createOkCancelButton(),
],
padding: EdgeInsets.all(10),
),
);
The result looks like the below.
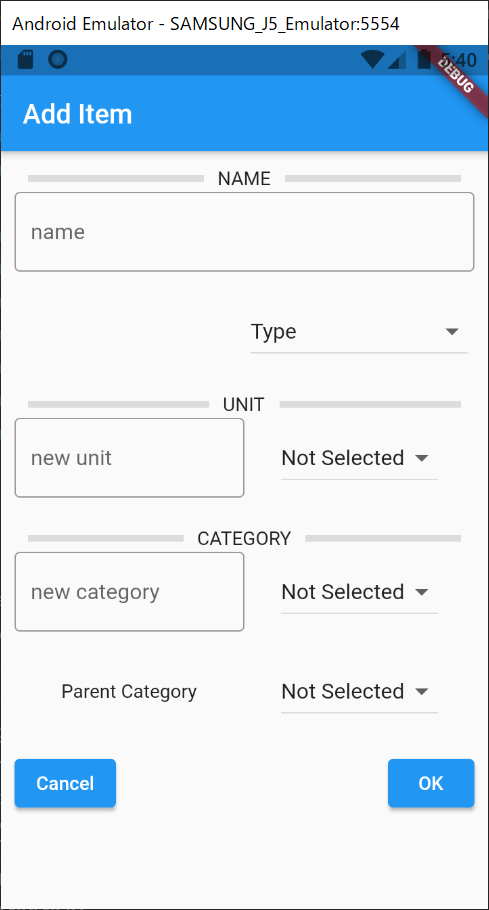
I haven’t understood yet why Expanded is needed but Divider doesn’t appear without it. The view looks like this without Expanded widget.
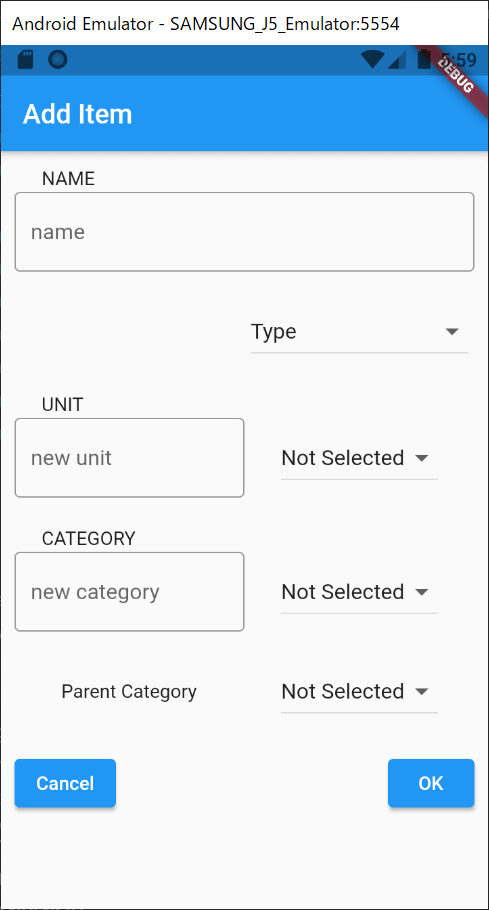
Comments